Table Of Content
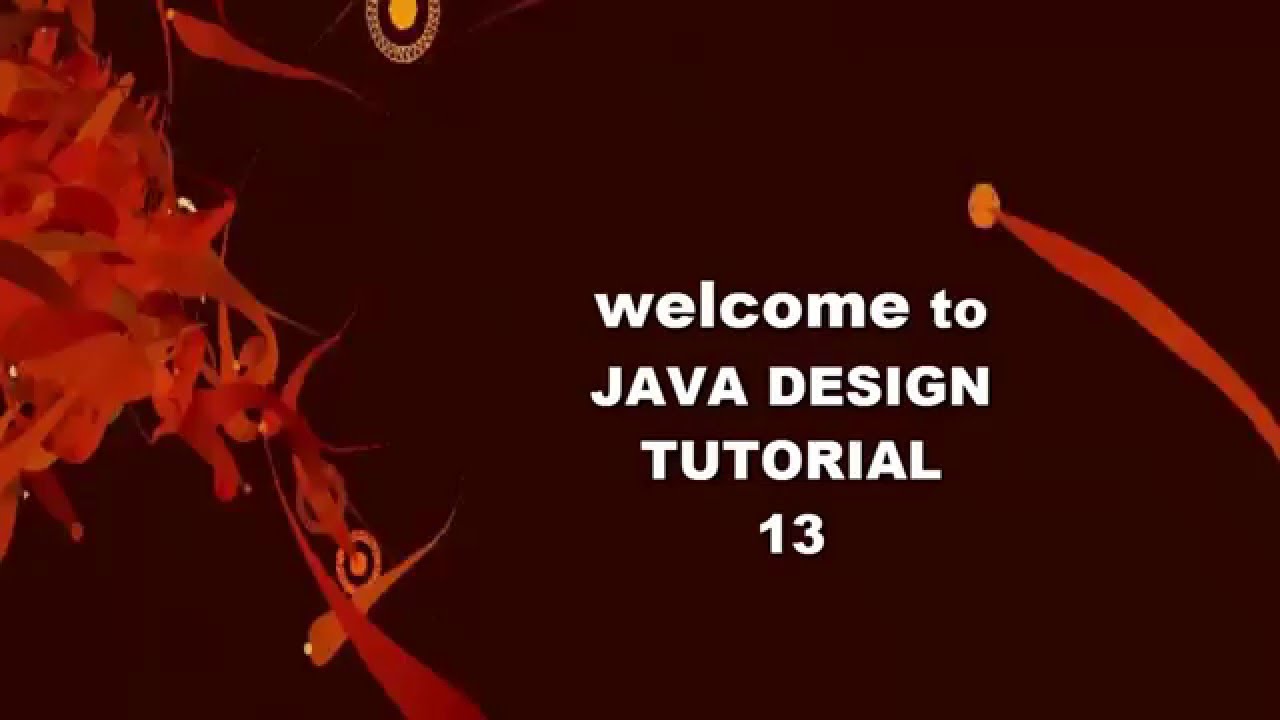
The chain of responsibility pattern is used to achieve loose-coupling in software design where a request from the client is passed to a chain of objects to process them. Then the object in the chain will decide who will be processing the request and whether the request is required to be sent to the next object in the chain or not. Structural design patterns provide different ways to create a Class structure (for example, using inheritance and composition to create a large Object from small Objects). Facade Method is a structural design pattern, it provides a simplified, higher-level interface to a set of interfaces in a subsystem, making it easier for clients to interact with that subsystem. The alchemist must be able to create both gold and copper coins and switching between them must be possible without modifying the existing source code.
Step 5
This not only makes our architecture more flexible but also less fragile. The code that uses the factory method (often called the client code) doesn’t see a difference between the actual products returned by various subclasses. The client knows that all transport objects are supposed to have the deliver method, but exactly how it works isn’t important to the client. Important to note that the Factory design pattern is one of the most used Java design patterns for creating objects. The client will get newly instantiated objects using a common interface. Factory pattern is most suitable where there is some complex object creation steps are involved.
Factory Method Pattern
In the example provided, Currency is the product interface with the method getSymbol(). Let’s consider the above-mentioned diagram for implementing a factory design pattern. Here is a Factory Method pattern to convert currency values between Indian Rupees (INR), US Dollars (USD), and Great British Pounds (GBP). Each object is created through a factory method available in the factory - which can either be an interface or an abstract class. Car is parent class of all car instances and it will also contain the common logic applicable in car making of all types. AccountFactory is a class that implements the Factory design pattern.
đź’ˇ Why Use the Factory Design Pattern?
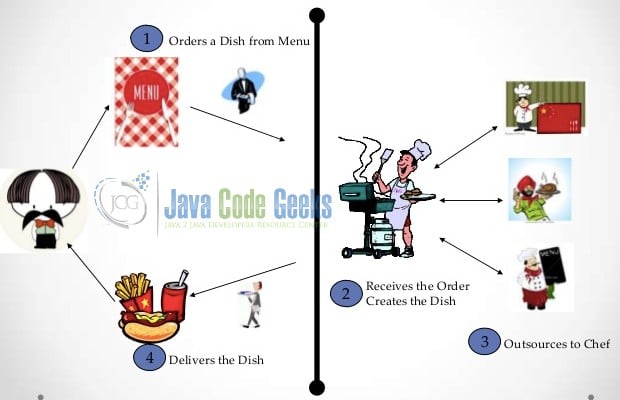
Enumeration below represents types of coins that we support (GoldCoin and CopperCoin). Buy the eBook Dive Into Design Patterns and get the access to archive with dozens of detailed examples that can be opened right in your IDE. Let’s imagine you are given a task to build an SMS notification service that notifies some users. As the initial requirements were just to build a simple SMS notifications class that contains business logic along with other information.
Saigon Technology, with its expertise in Java development, provides robust solutions that can help streamline your software development process. Lets you fit more objects into the available amount of RAM by sharing common parts of state between multiple objects instead of keeping all of the data in each object. Provides a simplified interface to a library, a framework, or any other complex set of classes. Lets you split a large class or a set of closely related classes into two separate hierarchies—abstraction and implementation—which can be developed independently of each other. This is because the proper implementation for calcPrice() is already present in the parent class. The visitor pattern is used when we have to perform an operation on a group of similar kinds of objects.
Product
The Factory design pattern provides a solution by encapsulating the object creation process in a separate factory class. By delegating the responsibility of object creation to a factory, the pattern promotes loose coupling, enhances flexibility, and simplifies maintenance. In this article, we will explore the fundamental principles, advantages, and potential disadvantages of the Factory design pattern, emphasizing its significance in object creation and encapsulation. Factory design pattern provides a way to create objects without specifying their exact class at compile time. It involves defining an interface or abstract class for creating objects (the factory) and allowing subclasses to alter the type of objects that will be created.
In the factory pattern , the factory class has an abstract method to create the product and lets the sub classes to create the concrete product. Also the examples you mentioned as being present in JDK as example of factory method is also not pure factory pattern. I want you to edit your post so as Correct information reaches the readers. It is one of the best ways to create an object where object creation logic is hidden from the client. Provides an interface for creating objects in a superclass, but allows subclasses to alter the type of objects that will be created.

Therefore it’s easier to extend the product construction code independently from the rest of the code. Of course, you can apply this approach to other UI elements as well. However, with each new factory method you add to the Dialog, you get closer to the Abstract Factory pattern. This example illustrates how the Factory Method can be used for creating cross-platform UI elements without coupling the client code to concrete UI classes.
All the code additions are similar to those done for the Petrol.java file. In our example, we’ll create a food ordering system that allows customers to order different types of dishes, such as pizza and sushi, using the Factory Pattern. The interpreter pattern is used to define a grammatical representation of a language and provides an interpreter to deal with this grammar. The proxy pattern provides a placeholder for another Object to control access to it. This pattern is used when we want to provide controlled access to functionality.
Factory Method Design Pattern define an interface for creating an object, but let subclass decide which class to instantiate. The company can define an abstract class called “Fuel” that represents the common features of all cars, such as the price. Then, it can create concrete subclasses for each type of fuel, like “Petrol”, “Diesel”, and “CNG,” which extend the Fuel class. According to Gang of Four (GOF), the Factory Design Pattern states that A factory is an object which is used for creating other objects. In technical terms, we can say that a factory is a class with a method.
The NotificationFactory class is responsible for constructing/creating objects for different types of notifications. The factory typically has a single method called getTypeName() with the parameters you'd wish to pass. Then, through as many if statements required, we check which exact class should be used to serve the call. The Factory Method separates product construction code from the code that actually uses the product.
String pool implementation in Java is one of the best examples of flyweight pattern implementation. State Method is a Behavioral Design Pattern, it allows an object to alter its behavior when its internal state changes. Momento Method is a Behavioral Design Pattern, it provide to save and restore the previous state of an object without revealing the details of its implementation.
Top Golang Interview Questions You Must Be Prepared For - Simplilearn
Top Golang Interview Questions You Must Be Prepared For.
Posted: Tue, 27 Feb 2024 08:00:00 GMT [source]
Proxy Method is a structural design pattern, it provide to create a substitute for an object, which can act as an intermediary or control access to the real object. As mentioned above the superclass in the Factory design pattern can be interfaces, abstract classes, or non-final classes. Let’s take an example of Bank Accounts like Personal, Business, and Checking and use the BankAccountFactory to create these accounts as per the client’s requirement. We will first see the superclass which in our case is an interface BankAccount, this interface will have a single method registerAccount(). Each concrete product provides its own implementation of the getSymbol() method. Base dialog works with products using their common interface, that’s why its code remains functional after all changes.
And it must all be put into a single place so that you don’t pollute the program with duplicate code. Use the Factory Method when you want to provide users of your library or framework with a way to extend its internal components.
When we need to create a structure in a way that the objects in the structure have to be treated the same way, we can apply the composite design pattern. The singleton pattern restricts the instantiation of a Class and ensures that only one instance of the class exists in the Java Virtual Machine. The implementation of the singleton pattern has always been a controversial topic among developers. A design pattern is a well-described solution to a common software problem. A design pattern is a generic repeatable solution to a frequently occurring problem in software design that is used in software engineering. It is a description or model for problem-solving that may be applied in a variety of contexts.
No comments:
Post a Comment